Important note: The Computer Graphics coursework
MUST be submitted
electronically via CATE. For the deadline of the coursework see CATE.
Coursework
Before reading further please read the description of the environment and
data formats below!
The goal of the coursework is to produce a realistic looking 3D rendering
of a face. You need to solve ALL THREE of the following tasks:
- Produce and submit three different renderings which show the face
rendered using Gouraud shading. You should use different view points and
lighting conditions for all three renderings. One of the renderings should
have a view point and lighting which is as similar as possible to the
renderings shown below.
- Produce and submit one rendering with a texture mapping
(see below for details about the texture map and texture coordinates in the
data files)
- Produce and submit two animations: In the first
animation you should rotate the camera 360 degrees around the face. In the
second animation zoom in and out of the face. Both animations should contain
at least 20 frames and should be saved as animated gifs (you can use various
tools to create animated gifs, for example ImageMagick or gifmerge).
You also need to submit your source code (a tar/zip file) which you wrote
to produce the renderings.
Description
The coursework for the graphics course are practical programming exercises
which should be done using OpenGL. The task is to generate different
visualizations of a surface model of a face using the data shown below:
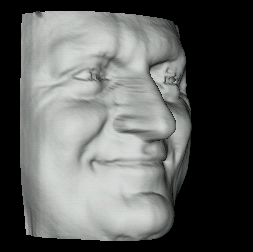
Environment
The programming environment for the exercises is OpenGL on the Linux/X11
machines of the department. OpenGL should be installed on all Linux/X11
machines. You can also use the Windows machines in the lab but I recommend
to use the Linux environment. You can program either in C or C++.
You can download a skeleton OpenGL program (for Linux) from here:
Create a new directory in your home directory and download all three files
into this directory. To compile the program, go to the new directory and
type make. This should compile the sample program for you. To run the sample
program, type ./cgRender. The skeleton OpenGL program should create an empty
window for you. Take a good look at the skeleton program. It contains a number
of hints on how to use OpenGL to start solving the coursework. If you have
questions about the coursework come to the tutorials!
Data
You can download the data file for an exisiting surface model of a face
from
here. This surface model consists of vertex
coordinates, polygon data and texture coordinates. You can assume that the
surface model contains only simple and planar polygons. In addition, you
can assume that the surface model is consistent, i.e. the ordering of
vertices of the polygons is always the same. The surface model is stored in
a file which has the following four parts:
- Header. The header contains the following lines:
# vtk DataFile Version 3.0
Somebody's face
ASCII
DATASET POLYDATA
- Vertex data. The first line contains the key word POINTS
to indicate the start of the vertex data. The next number equals the number
of vertices of the surface model, followed by the type of each vertex (you
can assume that this always corresponds to float). Each of the following lines contains
the x, y and z world-coordinates for each vertex as floating point numbers.
- Polygon data. The first line contains the key word POLYGONS to
indicate the start of the polygon data, followed by the number of polygons and
the size of the cell list for all polygons. Each of the following lines
contains the number of vertices forming the polygon, followed by the indices
of the vertices which form the polygon.
- Texture data. The first line will contain the key word CELL_DATA
followed by a number which you can ignore. The next line will contain
the keyword POINT_DATA, followed by the number of points
with texture coordinates. The next line contains the
key word TEXTURE_COORDINATES to indicate the start of
the texture data and some misc. information. After that follow lines containing
the x and y texture coordinates for each vertex as floating point numbers.
The order of the texture coordinates is the same as the order of the vertices.
You can download the data file for a 512 x 512 texture map of a face from
here. The texture maps are represented as PPM files
with a short ascii header followed by the R (red), G (green) and B (blue)
components for each pixel as a separate unsigned byte. To find out how the
typical header of a PPM file looks like try
man
ppm.